미로 탐색
1 초 | 192 MB | 108610 | 44808 | 28679 | 39.867% |
문제
N×M크기의 배열로 표현되는 미로가 있다.
1 | 0 | 1 | 1 | 1 | 1 |
1 | 0 | 1 | 0 | 1 | 0 |
1 | 0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 0 | 1 | 1 |
미로에서 1은 이동할 수 있는 칸을 나타내고, 0은 이동할 수 없는 칸을 나타낸다. 이러한 미로가 주어졌을 때, (1, 1)에서 출발하여 (N, M)의 위치로 이동할 때 지나야 하는 최소의 칸 수를 구하는 프로그램을 작성하시오. 한 칸에서 다른 칸으로 이동할 때, 서로 인접한 칸으로만 이동할 수 있다.
위의 예에서는 15칸을 지나야 (N, M)의 위치로 이동할 수 있다. 칸을 셀 때에는 시작 위치와 도착 위치도 포함한다.
입력
첫째 줄에 두 정수 N, M(2 ≤ N, M ≤ 100)이 주어진다. 다음 N개의 줄에는 M개의 정수로 미로가 주어진다. 각각의 수들은 붙어서 입력으로 주어진다.
출력
첫째 줄에 지나야 하는 최소의 칸 수를 출력한다. 항상 도착위치로 이동할 수 있는 경우만 입력으로 주어진다.
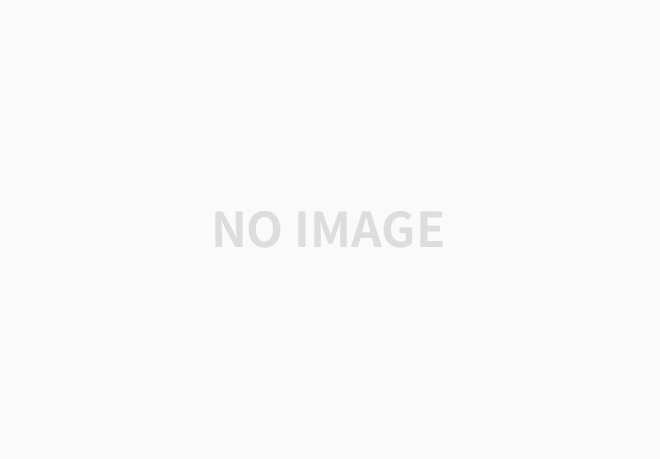
풀이1: 시간초과
이전과 같은 DFS방법을 사용했더니 시간초과가 일어났다.
import java.util.*;
public class Main {
static boolean[][] arr;
static boolean[][] check;
static int M,N;
static int min=10000;
public static void minTrack(int i,int j,int count) {
if(i<0 || i>=M || j<0 || j>=N || !arr[i][j] || check[i][j]) {
return;
}
count=count+1;
if(i==0 && j==0) {
min=Math.min(min, count);
}
check[i][j]=true;
minTrack(i+1,j,count);
minTrack(i-1,j,count);
minTrack(i,j+1,count);
minTrack(i,j-1,count);
check[i][j]=false;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
M=sc.nextInt();
N=sc.nextInt();
arr=new boolean[M][N];
check=new boolean[M][N];
for(int i=0;i<M;i++) {
String s = sc.next();
for(int j=0;j<N;j++) {
if(s.charAt(j)=='1') {
arr[i][j]=true;
}
}
}
minTrack(M-1,N-1,0);
System.out.println(min);
}}
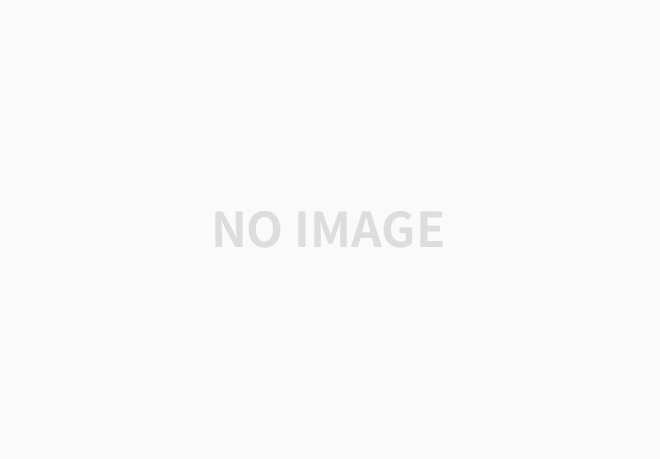
풀이2
BFS를 사용해 풀이한다. 두 가지를 주의해야한다.
1) x,y좌표를 객체형태로 넣어야하고 이를 위해 큐 자료형 또한 객체 배열을 넣을 수 있도록 한다.
Queue<int[]> queue = new LinkedList<int[]>();
queue.add(new int[] {x,y});
2) BFS는 DFS와 달리 상위 노드부터 차례차례 탐색하기 때문에 특정 위치에 먼저 도착하면 곧 최소 경로로 인정된다.
이로 인해: 다음항 = 이전항 + 1로 누적하더라도 값이 덮어씌워지지 않으며 도착점의 값을 구하면 최소 값을 구할 수 있다.
import java.util.*;
public class Main {
static int[][] arr;
static boolean[][] check;
static int M,N;
static int[] dx = {-1,1,0,0};
static int[] dy = {0,0,1,-1};
public static void bfs(int x, int y) {
Queue<int[]> queue = new LinkedList<int[]>();
check[0][0]=true;
queue.add(new int[] {x,y});
while(!queue.isEmpty()) {
int[] now = queue.poll();
int nowX = now[0];
int nowY = now[1];
for(int i=0;i<4;i++) {
int nextX = nowX + dx[i];
int nextY = nowY + dy[i];
if(nextX<0 || nextY<0 || nextX>=M || nextY>=N || arr[nextX][nextY]==0 || check[nextX][nextY]) {
continue;
}
queue.add(new int[] {nextX,nextY});
arr[nextX][nextY]=arr[nowX][nowY]+1;
check[nextX][nextY]=true;
}
}
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
M=sc.nextInt();
N=sc.nextInt();
arr=new int[M][N];
check=new boolean[M][N];
for(int i=0;i<M;i++) {
String s = sc.next();
for(int j=0;j<N;j++) {
if(s.charAt(j)=='1') {
arr[i][j]=1;
}
}
}
bfs(0,0);
System.out.println(arr[M-1][N-1]);
}}

'Study > Baekjoon' 카테고리의 다른 글
Baekjoon7569: 토마토 (0) | 2022.01.14 |
---|---|
Baekjoon7576: 토마토 (0) | 2022.01.14 |
Baekjoon1012: 유기농 배추 (0) | 2022.01.12 |
Baekjoon2667: 단지번호붙이기, 오답? (0) | 2022.01.11 |
Baekjoon2606: 바이러스 (0) | 2022.01.10 |